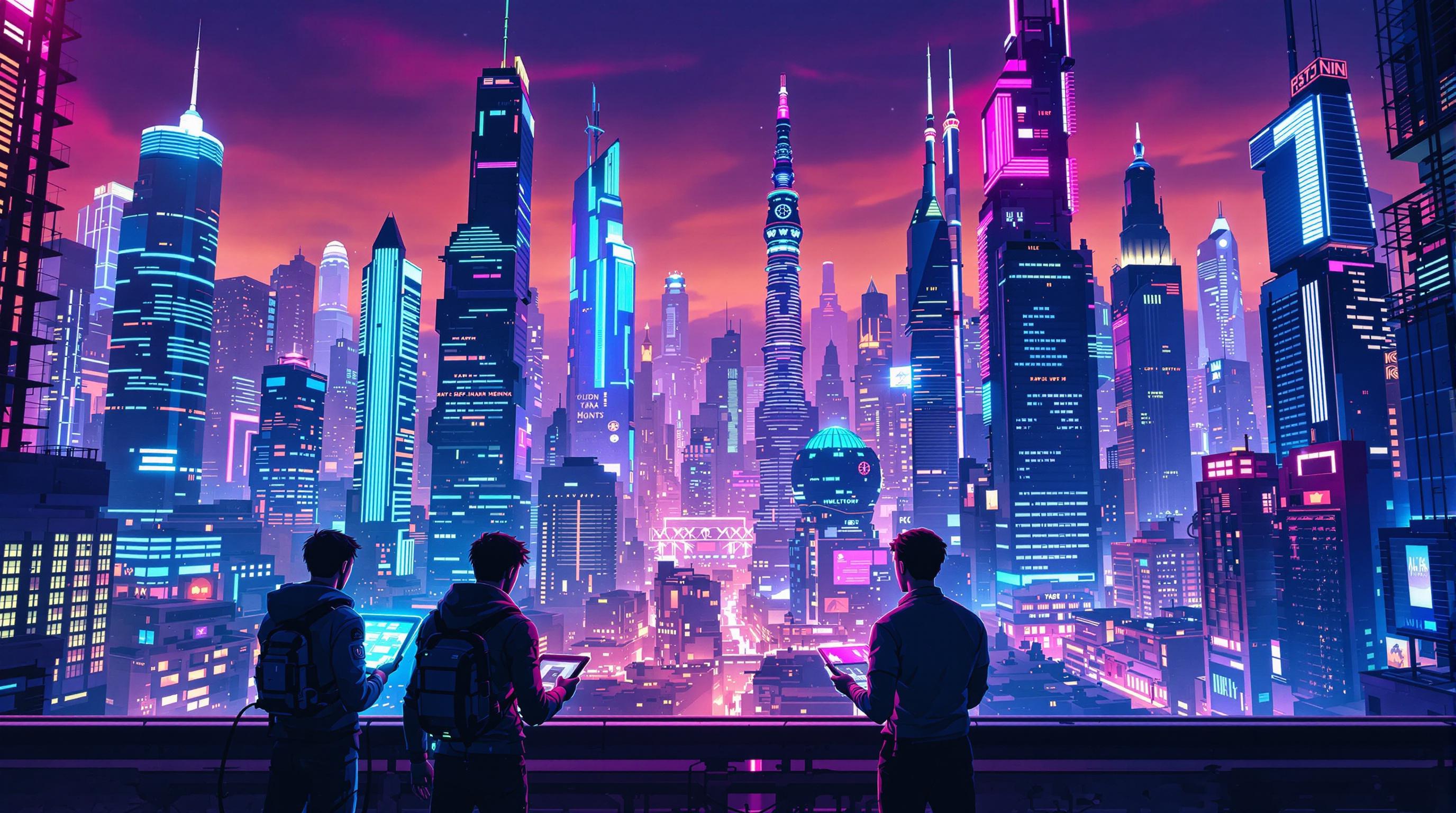
7 Common Web Scraping Errors and Solutions
7 Common Web Scraping Errors and Solutions
Web scraping can be tricky, but knowing how to handle common errors will make your data collection smoother. Here's a quick guide to the 7 most frequent web scraping issues and how to fix them:
- HTTP 429 (Too Many Requests): Add delays between requests and use rotating proxies.
- HTTP 403 (Access Denied): Use realistic user agents and headers.
- 5XX Server Errors: Implement a backoff strategy and retry mechanism.
- Dynamic Content: Use browser automation tools or specialized services.
- Missing Data: Employ detailed selectors and error handling.
- CAPTCHAs: Rotate IP addresses and mimic human behavior.
- Network Issues: Set up retry mechanisms and handle proxy errors.
Key tips to prevent errors:
- Rotate proxies smartly
- Act like a human user
- Follow website rules
- Use scraping APIs for tough sites
- Monitor your scraper's performance
Related video from YouTube
Common HTTP Status Errors
Let's talk about the HTTP status errors you'll run into when web scraping. Here's how to handle the big ones:
429 Error: Too Many Requests
This error is the server's way of saying "slow down!" You've sent too many requests too quickly.
To fix this:
1. Add delays
Slow down your requests. In Python, it's simple:
import time
time.sleep(5) # Wait 5 seconds between requests
2. Use rotating proxies
Spread your requests across different IP addresses. This keeps you from overwhelming a single server.
403 Error: Access Denied
A 403 error means the server understood you, but won't let you in. Often, it's because the site caught on to your scraping.
Here's how to get past it:
1. Use a realistic user agent
Websites can spot default scraper user agents easily. Try this instead:
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/127.0.6533.122 Safari/537.36"
}
2. Flesh out your headers
Make your request look like it's coming from a real browser:
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:98.0) Gecko/20100101 Firefox/98.0",
"Accept": "text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,*/*;q=0.8",
"Accept-Language": "en-US,en;q=0.5",
"Accept-Encoding": "gzip, deflate",
"Connection": "keep-alive",
"Upgrade-Insecure-Requests": "1",
"Sec-Fetch-Dest": "document",
"Sec-Fetch-Mode": "navigate",
"Sec-Fetch-Site": "none",
"Sec-Fetch-User": "?1",
"Cache-Control": "max-age=0",
}
5XX Errors: Server Problems
5XX errors mean the problem is on the server's end, not yours. The 500 Internal Server Error is the most common.
When you hit these:
1. Wait it out
Sometimes, doing nothing is best. Give the server some time and try again later.
2. Retry carefully
Use a backoff strategy. Start with a short delay, then increase it if the error sticks around. Here's how:
import time
import random
def make_request(url, max_retries=3):
for attempt in range(max_retries):
try:
response = requests.get(url)
response.raise_for_status()
return response
except requests.exceptions.RequestException:
if attempt < max_retries - 1:
sleep_time = (2 ** attempt) + random.random()
time.sleep(sleep_time)
else:
raise
Data Extraction Problems
Web scraping isn't always a walk in the park. Even after you've tackled HTTP errors, you might hit some snags when trying to extract data. Let's look at some common hurdles and how to jump over them.
Loading Dynamic Content
Modern websites often use JavaScript to load content on the fly, which can trip up regular scrapers. Here's how to handle it:
1. Browser automation tools
Tools like Selenium, Playwright, or Puppeteer can run JavaScript and interact with web pages just like a real browser would.
This tool is built to handle dynamic content and complex interactions. It uses custom-built Chromium browsers that are tough to detect.
For instance, say you're scraping a product page that loads more items as you scroll. You could use StealthBrowser.cloud's API to mimic scrolling and grab all those dynamically loaded products.
Fixing Missing Data Issues
Missing data can throw a wrench in your scraping plans. Here's how to make sure you're getting the full picture:
- Use detailed selectors: Don't just rely on simple CSS selectors. Try specific XPath queries or mix and match selectors for better accuracy.
- Add error handling: Use try-except blocks to catch and log errors. This helps you spot where data's going missing and why.
- Check your data: Regularly look over your scraped data to make sure it's complete. Tools like pandas in Python can help you spot missing or weird values quickly.
Here's a real-world example from a scraping project:
import re
# Instead of:
# Phone.append(cells[5].find(text=True).replace('T: ',''))
# Try this:
Phone.append(re.search(r'(\d{3}-\d{3}-\d{4})', cells[5].get_text()).group())
This regex pattern makes sure you're grabbing the actual phone number, even if the HTML structure isn't consistent.
Dealing with CAPTCHAs
CAPTCHAs are designed to block automated access, making them a real headache for web scrapers. Here's how to work around them:
- Switch up your IP addresses: Use good proxy networks to spread out your requests and avoid triggering CAPTCHAs.
- Act more human-like: Add random delays between requests and change your user agents to seem less bot-like.
- Try CAPTCHA solving services: If CAPTCHAs keep popping up, you might need to use services like 2Captcha or Anti-CAPTCHA as a last resort.
- Use browser automation: Tools like StealthBrowser.cloud can help you get past CAPTCHAs by mimicking real browser behavior.
Remember, the goal is to scrape responsibly without overwhelming the website you're targeting. As one data scientist puts it:
"To handle incomplete data when web scraping, use try-except blocks to handle errors gracefully, implement retries with delays to address temporary issues, and prioritize robust error logging for later analysis."
Network Issues
Web scraping can hit snags when network problems pop up. Let's look at some common issues and how to fix them.
Connection Timeouts
Connection timeouts happen when a server takes too long to respond. Here's how to deal with them:
1. Slow Down Your Requests
If you're hitting the server too fast, add delays between requests:
import time
import requests
def make_request(url):
time.sleep(2) # 2-second pause
return requests.get(url)
2. Use a Retry Mechanism
Set up automatic retries for failed requests:
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
def requests_retry_session(retries=3, backoff_factor=0.3):
session = requests.Session()
retry = Retry(total=retries, backoff_factor=backoff_factor)
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
return session
# How to use it
session = requests_retry_session()
response = session.get('https://example.com')
This code retries up to 3 times, with increasing delays between attempts.
Proxy Errors
Proxies help avoid IP bans, but they can cause problems too. Here's how to handle them:
1. Pick Good Proxies
Not all proxies are the same. Bright Data offers high-quality residential proxies that websites are less likely to block.
2. Rotate Your Proxies
Don't stick to one proxy. Use a pool of proxies to lower the chances of getting caught:
import random
import requests
proxy_pool = [
'http://proxy1.example.com:8080',
'http://proxy2.example.com:8080',
'http://proxy3.example.com:8080'
]
def get_random_proxy():
return random.choice(proxy_pool)
url = 'https://example.com'
proxies = {'http': get_random_proxy()}
response = requests.get(url, proxies=proxies)
3. Handle Proxy Timeouts
Set a timeout for your requests in case a proxy is slow:
try:
response = requests.get(url, proxies=proxies, timeout=10)
except requests.exceptions.Timeout:
print("Request timed out")
Dealing with Network Latency
Network latency can slow down your scraper. Try these tactics:
1. Use Asynchronous Requests
Libraries like aiohttp
let you make multiple requests at once, speeding things up.
2. Pick Proxies Close By
If you're scraping a US website, use US-based proxies to cut down on latency.
3. Use Caching
For data that doesn't change often, cache it to reduce network requests.
Remember to be respectful of the websites you're scraping. As Aw Khai Sheng, an experienced web scraper, says:
"The road to smooth web-scraping is paved with exceptions - and learning how to deal with them!"
sbb-itb-45cd9a4
How to Prevent Errors
Web scraping can be tricky. But don't worry - with the right approach, you can dodge many common pitfalls. Let's look at some key strategies to keep your scraping smooth and error-free.
Smart Proxy Rotation
Want to avoid IP blocks and rate limiting? Use rotating proxies. It's one of the best ways to keep your scraping on track.
Here's a smart way to rotate proxies by subnet:
import random
proxies = ["xx.xx.123.1", "xx.xx.123.2", "xx.xx.123.3", "xx.xx.124.1", "xx.xx.124.2", "xx.xx.125.1", "xx.xx.125.2"]
last_subnet = ""
def get_proxy():
global last_subnet
while True:
ip = random.choice(proxies)
ip_subnet = ip.split('.')[2]
if ip_subnet != last_subnet:
last_subnet = ip_subnet
return ip
This code makes sure you're not using the same subnet back-to-back. It's a simple trick that can help you fly under the radar.
Act Like a Human
Websites are getting better at spotting bots. So, your scraper needs to act more human-like. Here's how:
Add some randomness to your requests. Don't fire them off rapid-fire style. Instead, add varying pauses between them:
import time
import random
def make_request(url):
response = requests.get(url)
sleep_time = random.uniform(1, 10)
time.sleep(sleep_time)
return response
And make your requests look like they're coming from a real browser:
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Referer": "https://www.google.com/",
"Accept-Language": "en-US,en;q=0.9",
"Accept-Encoding": "gzip, deflate, br"
}
Play by the Rules
Before you start scraping, always check the website's robots.txt
file. It's like a rulebook that tells you which parts of the site you can scrape. Ignore it, and you might find yourself blocked faster than you can say "IP ban".
Use a Scraping API
Some websites are tough nuts to crack. For these, you might want to use a scraping API. Services like ZenRows or ScraperAPI can handle complex anti-bot measures for you.
Here's what Raluca Penciuc, an expert at WebScrapingAPI, says:
"Residential proxies combined with an IP rotation system and a script that cycles request headers (especially user-agent) provide the best cover."
Keep Your Eyes Open
Websites change, and your scraper should too. Keep an eye on your scraping logs and set up alerts for failed attempts. This way, you can quickly adjust your strategy if a website updates its defenses.
Better Error Handling Methods
Errors in web scraping? They're part of the game. But with smart tactics, you can turn these hiccups into wins. Let's explore some advanced error handling techniques to beef up your scraping projects.
Smart Retry Mechanisms
Want to boost your scraper's success rate? Implement a smart retry mechanism. It's like giving your scraper a second (or third, or fourth) chance at bat.
Here's a Python example using requests
and urllib3
:
import requests
from requests.adapters import HTTPAdapter
from urllib3.util import Retry
retry_strategy = Retry(
total=4,
status_forcelist=[403, 429, 500, 502, 503, 504],
backoff_factor=0.1
)
adapter = HTTPAdapter(max_retries=retry_strategy)
session = requests.Session()
session.mount("http://", adapter)
session.mount("https://", adapter)
response = session.get('https://example.com')
This code sets up a retry strategy that gives each request four shots, with a 0.1-second pause between tries. It targets common HTTP error codes that often signal temporary glitches.
Exponential Backoff
Want to make your retry mechanism even smarter? Use exponential backoff. It's like taking a longer breather after each failed attempt.
The math behind it:
backoff_time = backoff_factor * (2 ** (current_retry - 1))
This approach mimics human behavior, making your scraper less likely to trip bot alarms.
Custom Error Handling
Generic error handling is okay, but custom error handling? That's where it's at. By creating specific exception classes, you can tackle each error type head-on.
Check this out:
class ParseError(Exception):
pass
class RateLimitError(Exception):
pass
try:
# Your scraping code here
except ParseError:
# Handle parsing errors
except RateLimitError:
# Handle rate limiting
except Exception as e:
# Handle other exceptions
This way, you can tailor your response to each specific error, making your scraper more reliable.
Logging and Monitoring
Catching errors is great, but understanding them? Even better. Set up a solid logging system to keep tabs on what's going wrong.
Here's a simple example using Python's logging
module:
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
try:
# Your scraping code here
except Exception as e:
logger.error(f"An error occurred: {str(e)}", exc_info=True)
For big scraping projects, consider using a tool like Sentry for real-time error tracking. It's like having a watchdog for your scraper.
Handling Dynamic Content
Modern websites love their JavaScript. To handle this dynamic content, you might need a headless browser like Selenium or Puppeteer.
Here's a quick Selenium example:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_argument("--headless")
driver = webdriver.Chrome(options=chrome_options)
driver.get("https://example.com")
content = driver.page_source
driver.quit()
This approach lets you interact with the page like a real browser, ensuring you can grab all the content, even the stuff loaded dynamically.
As web scraping expert Uday Shadani says:
"To handle these errors, you can use a try-except block to catch the error and handle it appropriately."
Smart error handling isn't just about fixing problems - it's about making your scraper more resilient and efficient. Give these techniques a shot and watch your scraping success rate soar!
Conclusion
Web scraping can be a game-changer for data collection, but it's not without its hurdles. Let's recap how to boost your scraping success and dodge common pitfalls:
1. Tackle HTTP errors like a pro
Don't let status errors trip you up. Use smart retry tactics with exponential backoff for those pesky 429 and 403 errors. It's all about acting more human-like to fly under the radar of anti-bot systems.
2. Conquer dynamic content
JavaScript-heavy sites giving you a headache? Selenium or Playwright are your new best friends. As web scraping guru Farhan Ahmed puts it:
"From my testing, Selenium Stealth works best for avoiding bot detection."
3. Play IP musical chairs
Dodge IP bans and rate limits by rotating your IPs and using proxies. High-quality residential proxies from services like Bright Data can seriously up your scraping game.
4. Play by the rules
Always check the robots.txt file and stick to the site's terms of service. It's not just about ethics - it's about keeping the peace with website owners.
5. Error-proof your scraper
Use try-except blocks, custom error classes, and thorough logging. It's like giving your scraper a bulletproof vest - it'll keep running smoothly even when things go sideways.
6. Be more human
Mix up your request timing, use realistic headers, and consider antidetect browsers. The goal? Make your scraper blend in with the crowd.
7. Keep your data squeaky clean
Regular checks and audits are key to maintaining top-notch data quality.
Remember, great web scraping isn't just about grabbing data - it's about doing it right. As PromptCloud wisely notes:
"Understanding and respecting these legal and ethical guidelines is essential for conducting responsible and sustainable web scraping practices."
So there you have it - your roadmap to smarter, smoother web scraping. Now go forth and scrape responsibly!
FAQs
What is a scrape error?
A scrape error is a hiccup you'll face when trying to grab data from websites. These pesky problems can be anything from simple setup mistakes to tricky site-specific issues that'll make you scratch your head.
Here's what the folks at ScrapeHero say about it:
"Web scraping errors are obstacles that every data enthusiast encounters. They can range from simple misconfigurations to complex site-specific issues. These errors often reveal the delicate nature of web scraping, where small changes can have significant impacts."
Scrape errors show just how fragile web scraping can be. One tiny change in a website's setup or security, and boom - your data collection hits a wall. That's why you've got to stay sharp and keep tweaking your scraping game.
The Axiom Team, who know a thing or two about web scraping, put it this way:
"Solving these is part science, part art."
This hits the nail on the head. Fixing scrape errors isn't just about coding skills. It's about getting how the web works and coming up with clever ways to navigate it. You've got to be part tech whiz, part creative genius to crack these nuts.