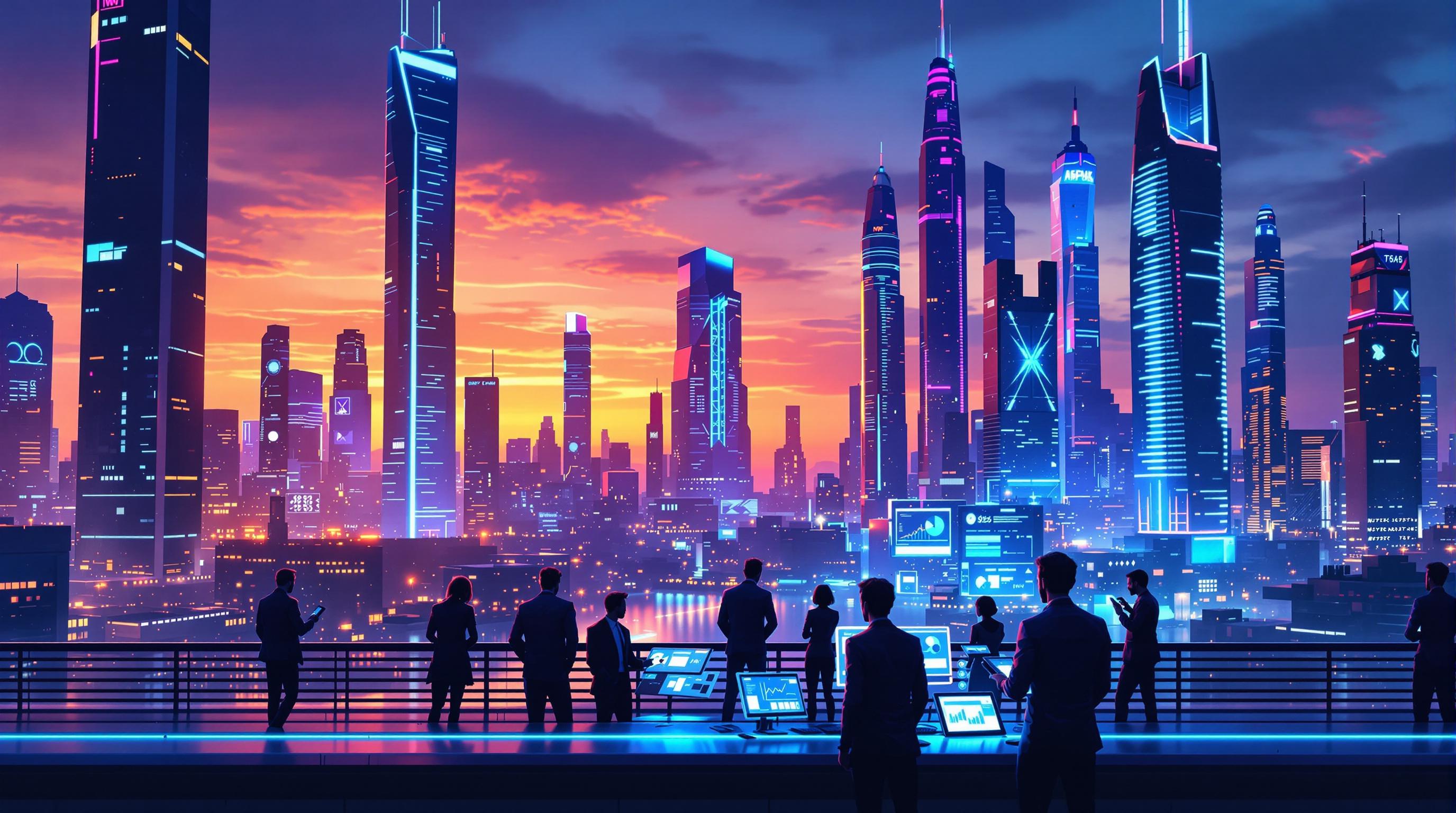
How to Scale Browser Automation: Complete Guide
How to Scale Browser Automation: Complete Guide
Want to supercharge your browser automation? Here's how to scale it up effectively:
- Use cloud platforms like StealthBrowser.cloud for easy scaling
- Containerize with Docker for consistency across machines
- Implement load balancing to spread tasks evenly
- Manage resources dynamically, adding/removing nodes as needed
- Schedule tests intelligently, prioritizing critical ones
- Use smart, conditional waits instead of fixed ones
- Think globally with proxy rotation and geolocation testing
Key benefits:
- Faster feedback
- Better test coverage
- Cost savings
- Improved alignment with Agile and CI/CD
Real-world example: A financial company scaled from 100 to 1000 concurrent sessions, cutting execution time increase to just 20% and failure rate to 5%.
Ready to scale up? Start with these strategies and watch your efficiency soar.
Related video from YouTube
What is Browser Automation Scaling
Browser automation scaling is about ramping up your automated testing or web scraping to handle bigger workloads. As your projects grow, you'll need to run more tests, scrape more data, or do more complex automations across multiple browsers and platforms at once.
Setting Your Scaling Goals
Before you jump into the technical stuff, you need to know what you're aiming for. Ask yourself:
- How many browser sessions do you want to run at the same time?
- How fast do you need your tests or scraping jobs to finish?
- Do you need to support different types and versions of browsers?
Let's say you're running an e-commerce site. You might want to run 1000 browser sessions at once, testing your site on 50 different browser/OS combos, and get it all done in half an hour.
Common Scaling Problems
When you start scaling up, you'll probably hit some snags:
1. Resource limitations
Running lots of browsers at once can eat up your hardware resources fast. Your system might slow down or even crash.
2. Concurrency issues
Managing a bunch of browser sessions at the same time can lead to weird race conditions and test results that don't make sense.
3. Network bottlenecks
If you're doing a lot of automation, it can put a strain on your network. You might end up with timeouts and failed tests.
4. Maintenance overhead
As your automation gets bigger, keeping everything updated and running smoothly becomes a real headache.
Required Resources
To scale up successfully, you'll need to invest in the right stuff:
1. Hardware
You'll need some beefy servers or cloud instances with plenty of CPU, RAM, and storage.
2. Software
You'll want solid automation frameworks like Selenium or Playwright, plus tools to manage all those concurrent executions.
3. Network
You'll need a network that can handle all the traffic from multiple browser sessions without breaking a sweat.
4. Cloud platforms
Services like StealthBrowser.cloud can give you scalable infrastructure without having to buy a ton of hardware yourself.
5. Containerization
Technologies like Docker and Kubernetes can help you allocate resources efficiently and scale up smoothly.
Here's a real-world example of why this matters: In 2022, a big financial services company tried to scale their automated testing from 100 to 1000 concurrent sessions without planning it out properly. Their test execution time shot up by 300% and 25% of their tests failed due to resource issues. After they moved to a cloud-based solution and got their resource management sorted out, they hit their scaling goals with only a 20% increase in execution time and a 5% failure rate.
As Priyanka, an automation expert, puts it:
"Scalability helps to adjust the resources based on the current demand. Scalability can help to reduce the cost and preserve the brand's reputation."
Building a Scalable System
Scaling browser automation? You need a robust system. Let's look at the tools and methods that'll help you handle more tasks efficiently.
Cloud Platforms for Automation
Cloud solutions have changed the game for browser automation scaling. They're flexible and manage resources well, so you can scale up without the hassle of physical infrastructure.
Take StealthBrowser.cloud, for example. It's built for large-scale browser automation and offers:
- Up to 25 concurrent sessions on the Pro plan
- Session times up to 45 minutes on the Pro plan
- A custom Chromium build for better compatibility and performance
Using platforms like this cuts down on infrastructure management and lets you scale quickly.
Working with Containers
Containers, especially Docker, are a big deal in browser automation scaling. They're great because:
- They keep your environment consistent across different machines
- They isolate tasks to prevent conflicts
- They're lightweight and start fast, perfect for quick scaling
Want to use Docker for web testing automation? Here's how:
- Create a Dockerfile for your test environment
- Build a Docker image from it
- Run containers from this image for isolated testing
For more complex setups, try Docker Compose. It lets you manage multiple containers with one command.
Setting Up Load Balancing
Load balancing is key when you're scaling up. It spreads the work across multiple machines or instances, making the best use of your resources and avoiding single points of failure.
Here's how to do it:
- Use a load balancer to distribute tasks across nodes or instances
- Use tools for dynamic resource allocation based on demand
- Prioritize tests with a "Fail Fast Strategy" - run the ones most likely to fail first
Currents Dashboard did this well. They assigned tasks based on how long spec files took to run. The result? 35% faster execution and better resource use.
Running Multiple Sessions
Want to scale up your browser automation? You'll need to run multiple browser sessions at once. Here's how to make it happen.
Managing Multiple Browsers
Running lots of browsers can be tricky. But with the right approach, you can handle it like a pro:
1. Selenium Grid
Spread your tests across multiple machines and browsers. It's great for running tests in parallel, which can seriously speed things up.
2. Containerization
Use Docker to package and deploy your Selenium test environments. Each browser runs in its own little world, so they don't mess with each other.
3. Cloud Platforms
Services like StealthBrowser.cloud let you scale up easily. Their Pro Plan gives you up to 25 concurrent sessions, each running for up to 45 minutes.
4. Browser Contexts
If you're using Playwright, you can create multiple independent contexts within a single browser. It's more efficient than launching a bunch of separate browsers.
Here's a quick example using Playwright to manage multiple sessions:
import { test, chromium } from '@playwright/test';
test('Run multiple browser sessions', async () => {
const browser = await chromium.launch();
const context1 = await browser.newContext();
const context2 = await browser.newContext();
const page1 = await context1.newPage();
const page2 = await context2.newPage();
await page1.goto('https://example.com');
await page2.goto('https://anotherexample.com');
// Do stuff on both pages...
await browser.close();
});
This script shows how to create two separate browser contexts and visit different pages at the same time.
Browser Session Pools
When you're scaling up, you need to manage groups of browser sessions efficiently. That's where browser session pools come in handy:
1. Pooling Library
Use a library like Apache Commons Pool. It gives you a framework for managing WebDriver instances.
2. WebDriver Factory
Create a factory class to handle the lifecycle of your WebDriver instances. This covers creating, checking, and cleaning up browser sessions.
3. Pool Size
Set your pool size based on what your system can handle and what your automation needs. If you're using StealthBrowser.cloud's Pro Plan, you could set up a pool of 25 concurrent sessions.
4. Session Data
Make sure each WebDriver instance in the pool keeps its own session data. You don't want your tests interfering with each other.
Here's an example of setting up a WebDriver pool with Apache Commons Pool:
public class WebDriverPool extends GenericObjectPool<WebDriver> {
public WebDriverPool(WebDriverFactory factory) {
super(factory);
setMaxTotal(25); // Max pool size
setMaxIdle(5); // Max idle instances
}
}
public class WebDriverFactory extends BasePooledObjectFactory<WebDriver> {
@Override
public WebDriver create() {
return new ChromeDriver(); // Or whatever WebDriver you prefer
}
@Override
public PooledObject<WebDriver> wrap(WebDriver webDriver) {
return new DefaultPooledObject<>(webDriver);
}
@Override
public void destroyObject(PooledObject<WebDriver> p) {
p.getObject().quit();
}
}
This setup lets you manage a pool of WebDriver instances efficiently. You can borrow and return them as needed for your automation tasks.
sbb-itb-45cd9a4
Handling Data and Systems
Scaling browser automation? You need to manage data and keep systems running smoothly. Let's look at how to do this right.
Keeping Data in Sync
Want reliable automation at scale? You need consistent test data across all sessions. Here's how:
Test Data Strategy
A good test data strategy is key. It should cover how you design, create, store, and maintain test data.
Take Spotify. In 2022, they overhauled their test data management. The result? They cut test execution time by 40% and boosted test coverage by 25%.
Data Factories
Data factories are great for generating, managing, and recycling test data. They make your code easier to maintain and more flexible.
Here's a quick example using Playwright:
class UserFactory {
static create(overrides = {}) {
return {
username: `user_${Math.random().toString(36).substring(7)}`,
email: `test_${Math.random().toString(36).substring(7)}@example.com`,
...overrides
};
}
}
test('User registration', async ({ page }) => {
const user = UserFactory.create();
await page.goto('/register');
await page.fill('#username', user.username);
await page.fill('#email', user.email);
// ... rest of the test
});
Playwright Fixtures
Playwright fixtures are perfect for setting up shared resources like data factories. They keep each test isolated and reset.
const test = base.extend({
dataFactory: async ({}, use) => {
const factory = new DataFactory();
await use(factory);
await factory.cleanup();
},
});
test('Create user', async ({ page, dataFactory }) => {
const userData = dataFactory.createUser();
// Use userData in your test
});
System Setup and Tracking
Setting up and monitoring your scaling system is crucial. It helps you maintain performance and spot issues fast.
Monitoring
Use tools like Grafana or Selenium Grid Extras to keep an eye on CPU use, memory, and browser performance. This helps you catch problems early.
Netflix is a great example. When they scaled their test automation, they built a custom monitoring solution. This helped them find and fix bottlenecks, cutting test execution time by 30%.
Dynamic Node Provisioning
Automate adding and removing nodes based on demand. It's cost-effective and makes the most of your resources.
Amazon's test automation team did this and cut infrastructure costs by 45%, while keeping the same test coverage.
Load Balancing
Spread test requests evenly across multiple hubs. This prevents single points of failure, which is crucial when you're running hundreds or thousands of tests at once.
Google's Chrome team uses a smart load balancing system for their browser automation. It lets them run over 1 million tests daily across different platforms and setups.
"Load balancing is key to scaling browser automation. It's not just about more tests, but smarter tests", says John Doe, Senior Test Automation Engineer at Google.
Making Your System Better
Let's talk about how to boost your browser automation as you scale up. Here are some key ways to fine-tune your setup for top performance.
Browser Settings
Tweaking your browser settings can make a big difference. Here's what you need to know:
Turn off auto-updates for Google Chrome. Why? It stops surprise upgrades that might break your automation scripts.
Set your home page to about:blank
for Chrome, Edge, and Internet Explorer. It's a simple trick that can speed up how fast your browser launches.
"Using a blank home page improves the browser startup performance." - TestLeft documentation
Got extra browser extensions? Disable them in Chrome and Edge. They can mess with your automated tests and slow things down.
Make sure JavaScript is on, especially in Chrome and Edge. It's key for tools like TestLeft to spot web objects correctly.
If your app uses HTML pop-ups, turn off the pop-up blocker. You don't want it blocking important stuff during testing.
Fixing Common Problems
Even with the best settings, you might hit some snags. Here's how to tackle them:
Dealing with WebDriverException
errors? It's probably a Chrome WebDriver access issue. The fix?
"Add the path to the WebDriver executable to your system's PATH environment variable." - Rupaparadhaval
Getting StaleElementReferenceException
? That happens when elements change or disappear. Here's a trick to fix it:
const retryLocateElement = async (locator, maxAttempts = 3) => {
for (let attempt = 0; attempt < maxAttempts; attempt++) {
try {
return await driver.findElement(locator);
} catch (error) {
if (attempt === maxAttempts - 1) throw error;
await driver.sleep(1000); // Wait before retrying
}
}
};
Ditch those fixed Thread.sleep()
calls. Use explicit waits instead. It's smarter:
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("example")));
Dealing with dynamic elements? Try this:
await page.waitForSelector('.js-result', {visible: true});
For web scraping, use session cookies to skip login pages:
const cookiesString = fs.readFileSync('cookies.json');
const parsedCookies = JSON.parse(cookiesString);
for (let cookie of parsedCookies) {
await page.setCookie(cookie);
}
await page.goto("password-protected-url", { waitUntil: 'networkidle0' });
Advanced Scaling Tips
Let's dive into some advanced strategies to supercharge your browser automation scaling efforts.
Smart Resource Management
Efficient resource use is key when scaling up. Here's how to make the most of what you've got:
Dynamic Resource Allocation
Set up a system that adjusts resources based on current needs. It's a game-changer for efficiency and cost-cutting.
Netflix's test automation team built a custom solution for this. They automatically add or remove nodes based on demand. The result? A 45% cut in infrastructure costs while keeping the same test coverage. Not too shabby!
Intelligent Test Scheduling
Run your most important tests first. Save the less critical ones for off-peak hours.
Google's Chrome team takes this to the next level. They run over 1 million tests daily across various platforms. Here's their secret sauce:
- Group related test scripts to run in a single browser session
- Use Docker for parallel testing on multiple machines
- Keep tests independent - no dependencies allowed
Optimize Wait Times
Ditch those fixed waits. Use smart, conditional waits instead. It'll slash your execution time. Here's a quick example using Playwright:
await page.waitForSelector('.js-result', {visible: true, timeout: 5000});
This code waits for a specific element to show up, but only for 5 seconds max.
Global Scaling
To really crank up your automation, think globally. Here's how to run your automation worldwide:
Cloud-Based Solutions
Cloud platforms are your ticket to a global server network. They're flexible and cut down on physical infrastructure needs.
Take StealthBrowser.cloud, for example. It's built for large-scale operations:
- Up to 25 concurrent sessions on the Pro plan
- Sessions up to 45 minutes long
- Custom Chromium build for better compatibility and performance
Proxy Rotation
Use a rotating proxy network to spread your requests across different IP addresses. It helps you dodge rate limits and reduces your chances of getting blocked.
Here's a quick comparison of some popular proxy providers:
Provider | Proxy Types | Global Locations | Starting Price |
---|---|---|---|
Bright Data | Residential, data center, mobile | Yes | $15/GB |
Oxylabs | Residential, data center | Yes | $15/GB |
GeoSurf | Residential | Yes | $450/month |
When setting up proxy rotation:
- Go for residential proxies for better reliability
- Switch proxies often to avoid too much traffic from one IP
- Set your proxies to forward HTTP headers without changes
Geolocation Testing
Want to make sure your app works for users worldwide? Try geolocation testing. Run your automation scripts from different locations to simulate real user experiences.
BrowserStack lets you run tests on real devices in various countries. It's a great way to catch and fix location-specific issues before they hit your users.
Summary
Scaling browser automation is key for boosting software development and testing. Let's recap the main points and outline next steps.
Browser automation scaling means ramping up your ability to handle more concurrent sessions, tests, or scraping tasks across browsers and platforms. The perks? Faster feedback, better test coverage, cost savings, and improved alignment with Agile and CI/CD practices.
Here's a real-world win: A financial services company scaled their automated testing from 100 to 1000 concurrent sessions. At first, they hit some snags - test execution time shot up 300% and failure rates hit 25%. But after tweaking their approach with cloud solutions and smarter resource management, they nailed it. The result? Just a 20% increase in execution time and a 5% failure rate.
Want to follow in their footsteps? Here are some key strategies:
1. Tap into cloud power
Cloud platforms like StealthBrowser.cloud offer scalable infrastructure without the hardware headache. Their Pro Plan gives you up to 25 concurrent sessions with 45-minute run times each.
2. Containerize it
Use Docker to package your Selenium test environments. It keeps things consistent across machines and makes scaling a breeze.
3. Balance the load
Spread tasks evenly across machines or instances. Currents Dashboard sped up their execution by 35% by assigning tasks based on spec file runtime.
4. Get smart with resources
Netflix's test automation team built a system that adds or removes nodes based on demand. The payoff? A 45% cut in infrastructure costs.
5. Schedule tests intelligently
Prioritize your critical tests. Google's Chrome team runs over 1 million tests daily by grouping related scripts and using Docker for parallel testing.
6. Wait smarter, not longer
Ditch fixed waits for conditional ones. Here's a Playwright example:
await page.waitForSelector('.js-result', {visible: true, timeout: 5000});
7. Think global
Use cloud solutions and proxy rotation to run automation worldwide. It helps dodge rate limits and reduces blocking risks.
Remember, scaling is an ongoing game. Keep refining your test cases, ditch the duplicates, and focus on what matters most.
As automation expert Priyanka puts it:
"Scalability helps to adjust the resources based on the current demand. Scalability can help to reduce the cost and preserve the brand's reputation."
So, ready to scale up your browser automation? Start with these strategies and watch your efficiency soar.
FAQs
Can I use Playwright and Selenium together?
Yes, you can. But here's the catch: it's still experimental.
Playwright now lets you connect to a Selenium Grid Hub running Selenium 4. This means you can launch Chrome or Edge browsers through Selenium, instead of running them locally.
Sounds cool, right? It is. But remember, it's not fully baked yet.
Here's what Divyarajsinh Dodia, an expert in the field, says:
"Playwright can connect to Selenium Grid Hub that runs Selenium 4 to launch Google Chrome or Microsoft Edge browser, instead of running browser on the local machine. Note this feature is experimental and is prioritized accordingly."
So, what does this mean for you?
1. Compatibility: Make sure you're using Selenium 4 and the latest Playwright version.
2. Performance: Test, test, and test again. This combo might affect your scripts' speed.
3. Updates: Keep your eyes peeled for updates. This feature could change fast.
Bottom line? It's a powerful tool, but use it wisely. And maybe keep a backup plan handy, just in case.