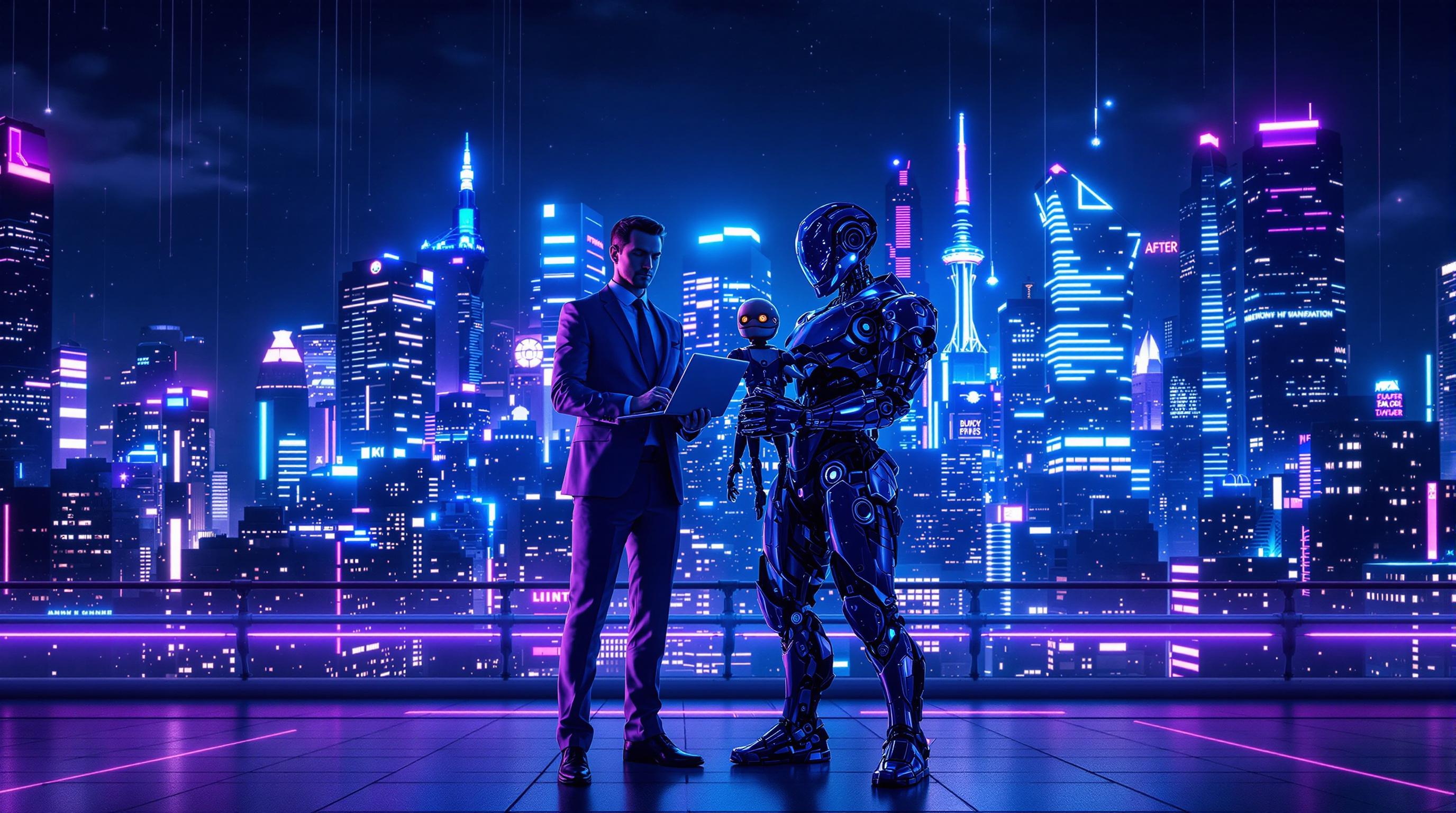
Playwright vs Puppeteer for Web Scraping
Playwright vs Puppeteer for Web Scraping
: Which Tool Fits Your Needs?
Choosing between Playwright and Puppeteer for web scraping? Here's what you need to know:
- Playwright: Multi-browser support, works with multiple programming languages
- Puppeteer: Chrome/Chromium specialist, JavaScript-focused
Quick Comparison:
Feature | Playwright | Puppeteer |
---|---|---|
Browser Support | Chrome, Firefox, WebKit | Mainly Chrome/Chromium |
Language Support | JavaScript, TypeScript, Python, Java, C# | JavaScript, TypeScript |
Best For | Complex, multi-browser tasks | Chrome-specific, simpler jobs |
GitHub Stars (Jan 2024) | 58k | 85.7k |
Key Takeaways:
- Playwright excels in cross-browser scenarios
- Puppeteer is faster for Chromium-specific tasks
- Both tools can use StealthBrowser.cloud for enhanced bot protection
- Your choice depends on project needs, team skills, and scalability requirements
Whether you're scraping millions of pages or focused on Chrome-based tasks, this guide will help you pick the right tool for your web scraping project.
Related video from YouTube
Key Features Compared
Playwright and Puppeteer each bring their A-game to web scraping. Let's break down their key features to help you pick the right tool for your project.
Supported Languages and Browsers
Playwright's got range. It works with JavaScript, TypeScript, Python, Java, and C#. This means you can stick with your favorite language and still use Playwright.
Puppeteer? It's all about JavaScript and TypeScript. But hey, that's not a bad thing - these languages are web dev staples.
When it comes to browsers, Playwright's like a Swiss Army knife:
Browser | Playwright | Puppeteer |
---|---|---|
Chromium | ✓ | ✓ |
Firefox | ✓ | Limited |
WebKit | ✓ | ✗ |
Playwright's multi-browser support is a big deal for cross-browser testing and scraping. Puppeteer's great with Chrome, but that's about it.
Speed and Resource Use
Both tools are speed demons, but they shine in different ways. Puppeteer's like a sprinter - super fast for Chrome-based tasks, especially in headless mode. It's perfect for quick scraping jobs.
Playwright's more of a decathlete. It handles multiple browsers without breaking a sweat. It's built to manage resources well, even when things get complicated.
Here's what Shanika Wickramasinghe from Frontend Weekly says:
"Overall, Playwright is ideal for testing complex web applications. Puppeteer is ideal for straightforward testing and web scraping tasks."
That pretty much sums up the performance trade-offs.
Working with Other Tools
How well a tool plays with others can make or break your project. Here's the lowdown:
Playwright comes with:
- Its own test runner (Playwright Test)
- Solid network interception
- Built-in mobile emulation
Puppeteer's got:
- Easy integration with Jest and Mocha
- Cool plugins like puppeteer-extra-plugin-stealth for ninja-level scraping
Playwright's built-in features give it an edge for complex stuff. But Puppeteer's simplicity and plugin ecosystem make it a favorite for straightforward scraping.
Both Playwright and Puppeteer work well with StealthBrowser.cloud. This combo gives you extra stealth powers, helping you avoid detection when scraping. StealthBrowser.cloud's custom Chromium builds work like a charm with both tools, adding an extra layer of invisibility to your scraping missions.
Web Scraping Tools and Methods
Playwright and Puppeteer are top-notch tools for web scraping. Let's explore how they can help you stay under the radar and handle sensitive data.
Avoiding Bot Blocks
Staying undetected is key for successful scraping. Both tools have tricks to help you dodge those annoying bot blocks.
Playwright comes with built-in stealth features. It automatically tweaks browser settings to make your scraper look more human. For example, it can mix up your User-Agent string, so each request seems to come from a different browser or device.
Here's how you can randomize User-Agent strings in Playwright:
const userAgentStrings = [
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/109.0.2227.0 Safari/537.36',
'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/109.0.3497.92 Safari/537.36',
// Add more User-Agent strings as needed
];
const randomUserAgent = userAgentStrings[Math.floor(Math.random() * userAgentStrings.length)];
await page.setUserAgent(randomUserAgent);
Puppeteer isn't as stealthy out of the box, but it has a secret weapon: the Puppeteer Stealth plugin. This add-on helps hide signs of automation, making your scraper blend in with normal web traffic.
"It's probably impossible to prevent all ways to detect headless chromium, but it should be possible to make it so difficult that it becomes cost-prohibitive or triggers too many false-positives to be feasible." - Puppeteer Stealth Documentation
To use Puppeteer Stealth, install it with Puppeteer Extra:
npm install puppeteer-extra puppeteer-extra-plugin-stealth
Both tools work well with proxies and adding delays between requests to mimic human browsing. This spreads your scraping traffic across different IP addresses, lowering the risk of getting blocked.
Managing Login Data
Handling login info safely is crucial when scraping sites that need authentication. Both tools offer ways to manage this sensitive data effectively.
Playwright has a neat feature called context.storageState()
. It lets you save and reuse login sessions, cutting down on repeated logins and making your scraping more efficient. Here's how to use it:
// Save login state
await context.storageState({ path: 'state.json' });
// Reuse login state in a new context
const context = await browser.newContext({ storageState: 'state.json' });
Puppeteer does something similar with its page.cookies()
method. You can save cookies after logging in and load them for later scraping sessions:
// Save cookies after login
const cookies = await page.cookies();
fs.writeFileSync('./cookies.json', JSON.stringify(cookies));
// Load cookies in a new session
const cookiesString = fs.readFileSync('./cookies.json');
const cookies = JSON.parse(cookiesString);
await page.setCookie(...cookies);
Both methods help you keep session states across scraping runs, making it less likely to trigger security alerts from logging in too often.
When working with login data, handle it carefully. Don't hardcode credentials in your scripts. Use environment variables or secure credential management systems to protect sensitive info.
Setup and Usage
Let's get you started with Playwright or Puppeteer for web scraping. Don't worry, it's not as tough as it might seem. We'll walk through the setup, where to find help, and how to use these tools with StealthBrowser.cloud for better bot protection.
Getting Started
Playwright and Puppeteer are both great, but they're built for different needs.
Playwright:
It works with multiple languages and browsers. Here's how to set it up:
- Make sure you have Node.js version 20 or higher.
- Open your terminal and run:
mkdir playwright-scraper && cd playwright-scraper
npm init -y
npm i playwright
- Now, let's test it out:
import { chromium } from 'playwright';
(async () => {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
await page.goto('https://github.com/topics/javascript');
await page.waitForTimeout(10000);
await browser.close();
})();
Puppeteer:
This one's all about Chromium browsers and JavaScript. Here's the setup:
- In your project folder, run:
npm init -y
npm install puppeteer
- Let's make sure it works:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: false });
const page = await browser.newPage();
await page.goto('https://github.com/topics/javascript');
await page.waitForTimeout(10000);
await browser.close();
})();
Both are easy to set up, but Playwright's multi-language support is a big plus for diverse teams.
Help and Resources
When you're stuck, here's where to look:
Playwright:
- Official docs
- GitHub repo
- Slack channel
Puppeteer:
- Official docs
- Stack Overflow
- GitHub Issues
Playwright's Slack channel is great for quick help, which can be a lifesaver on tight deadlines.
Using with StealthBrowser.cloud
Want to level up your scraping game? Here's how to use these tools with StealthBrowser.cloud:
- Get a StealthBrowser.cloud account and API key.
- Install the SDK:
For Playwright:
npm install playwright-extra playwright-extra-plugin-stealth
For Puppeteer:
npm install puppeteer-extra puppeteer-extra-plugin-stealth
- Update your script:
For Playwright:
import { chromium } from 'playwright-extra';
import stealth from 'playwright-extra-plugin-stealth';
chromium.use(stealth());
(async () => {
const browser = await chromium.connect({
wsEndpoint: `wss://cloud.stealthbrowser.com?token=YOUR_API_KEY`
});
// Your scraping code goes here
})();
For Puppeteer:
const puppeteer = require('puppeteer-extra');
const StealthPlugin = require('puppeteer-extra-plugin-stealth');
puppeteer.use(StealthPlugin());
(async () => {
const browser = await puppeteer.connect({
browserWSEndpoint: `wss://cloud.stealthbrowser.com?token=YOUR_API_KEY`
});
// Your scraping code goes here
})();
Using StealthBrowser.cloud gives you access to Chromium browsers that are built to dodge fingerprinting and other detection tricks. This setup makes your web scraping more reliable and scalable, especially when you're dealing with websites that have tough bot detection.
sbb-itb-45cd9a4
Advanced Features
Playwright and Puppeteer pack some serious firepower for complex web scraping. Let's see how they handle multiple tasks and location/privacy settings.
Running Multiple Tasks
Playwright is a beast when it comes to juggling multiple scraping jobs. It manages resources like a pro, letting you scale up without breaking a sweat.
Here's a mind-blowing fact: Apify scraped 90 million web pages with Puppeteer in just two months. But they found Playwright even more efficient for big jobs, thanks to its multi-browser support and isolated contexts.
Playwright's secret weapon? Multiple browser contexts in a single instance. It's like having superpowers for parallel scraping. Check this out:
const browser = await chromium.launch();
const context1 = await browser.newContext();
const context2 = await browser.newContext();
const page1 = await context1.newPage();
const page2 = await context2.newPage();
// Scrape away on page1 and page2 at the same time
Puppeteer can handle multiple tasks too, but it might struggle with parallel ops, especially in Firefox. It's built for Chrome and Chromium, which can be a bit limiting.
Location and Privacy Settings
Both tools have tricks up their sleeves for managing location data and staying under the radar, but Playwright has a slight edge.
Playwright makes proxy setup a breeze:
const browser = await chromium.launch({
proxy: {
server: 'http://myproxy.com:3128',
username: 'user',
password: 'pass'
}
});
Puppeteer can do proxies too, but it takes a bit more work:
const browser = await puppeteer.launch({
args: ['--proxy-server=http://myproxy.com:3128']
});
// Set up authentication if needed
await page.authenticate({
username: 'user',
password: 'pass'
});
When it comes to staying incognito, both tools have their strengths. Playwright lets you switch between Chrome, Firefox, and WebKit, making it harder for websites to spot your bot.
Lukáš Křivka from Apify drops this knowledge bomb:
"If blocking is a problem, Firefox can help by making it unnecessary to use residential proxies."
Puppeteer users, don't worry - the Puppeteer Stealth plugin has your back. It helps your scraper blend in with the crowd.
Want extra protection? Try StealthBrowser.cloud with either tool. Their custom Chromium builds are like invisibility cloaks for your scraper.
Which Tool to Pick
Picking between Playwright and Puppeteer for web scraping isn't straightforward. Let's look at the key factors to help you choose.
Best Uses for Each Tool
Playwright excels in complex, multi-browser scenarios. Its cross-browser support is a big plus for teams scraping data across different browser engines. Apify, a web scraping platform, used Playwright to scrape 90 million web pages in just two months. How? Playwright can handle multiple browser contexts in one instance.
Puppeteer is best for Chromium-focused projects. It's great for simpler scraping tasks. Take Ahrefs, an SEO tool. They use Puppeteer for their site audit feature, which crawls websites for SEO issues. Puppeteer's Chromium optimization makes it faster for these tasks.
Here's a quick comparison:
Feature | Playwright | Puppeteer |
---|---|---|
Browser Support | Chrome, Firefox, WebKit | Mainly Chrome/Chromium |
Language Support | JavaScript, TypeScript, Python, C#, Java | JavaScript, TypeScript |
Learning Curve | Steeper (more features) | Easier for JavaScript devs |
Performance | Great for complex, multi-browser tasks | Faster for Chromium-specific tasks |
Team Skills and Costs
Your team's skills and budget matter too.
If your team knows multiple programming languages, Playwright's support for JavaScript, Python, C#, and Java is a big win. It can lead to faster adoption across different projects.
Both tools are open-source, but the real costs come from development time and infrastructure. Playwright might need more upfront investment in learning and setup. But for big projects needing cross-browser compatibility, this can pay off with lower long-term costs and better efficiency.
Puppeteer, focusing on JavaScript and Chromium, can be cheaper for teams good at these technologies. Its simpler learning curve means faster setup for basic scraping tasks.
Here's a real example: Trivago, the hotel search platform, switched from Puppeteer to Playwright for testing. Despite the learning curve, they cut test execution time by 30% and reduced flaky tests. This led to big savings in their CI/CD pipeline.
Don't forget to factor in extra tools or services. If you're dealing with sites that have tough anti-bot measures, you might need a service like StealthBrowser.cloud. It works with both Playwright and Puppeteer, offering custom Chromium builds to bypass detection. This can be key for keeping your scraping operations running smoothly.
Summary
Picking between Playwright and Puppeteer for web scraping? It's all about what you need. Let's break it down:
Jack of All Trades or Master of One?
Playwright's your go-to for multi-browser action. It handles Chrome, Firefox, and WebKit like a champ. Perfect if you're juggling different browsers. Puppeteer? It's the Chromium specialist. Great for simpler scraping jobs.
Speaking Your Language
Got a polyglot team? Playwright's got you covered with JavaScript, TypeScript, Python, Java, and C#. Puppeteer keeps it simple with JavaScript and TypeScript only.
Bells and Whistles
Playwright comes loaded with cool stuff like auto-waiting and mobile emulation. Handy for tricky scraping jobs. Puppeteer keeps things lean but can zoom through Chromium tasks.
Real-World Wins
Take Trivago. They switched from Puppeteer to Playwright for testing and BAM! 30% faster test runs. That's some serious time (and cash) saved.
Strength in Numbers
As of January 2024, Puppeteer's rocking 85.7k GitHub stars. Playwright's not far behind with 58k. More stars often mean more help when you're stuck.
Staying Under the Radar
Both tools can team up with services like StealthBrowser.cloud to dodge bot detectors. Crucial when you're up against websites with tough anti-scraping defenses.
Bottom line? Think about what you need most: flexibility, speed, or specific features. That'll point you to your perfect scraping sidekick.
FAQs
Is Puppeteer still used?
You bet! Puppeteer's still going strong in 2024. With 85.7k GitHub stars and over 3 million monthly downloads, it's clear developers aren't ready to let go.
Why's it so popular? Puppeteer shines when it comes to Chrome and Chromium-based browsers. Take Ahrefs, for example. This big-name SEO tool uses Puppeteer to power their site audit feature. It crawls websites looking for SEO issues, and Puppeteer's Chrome optimization makes it super efficient for this kind of task.
Is Playwright better than Puppeteer in 2024?
It's not a clear-cut answer - it really depends on what you're trying to do.
Playwright's the new kid on the block, introduced by Microsoft in 2020. It's been turning heads because it works with Chrome, Firefox, and WebKit. If you need to test or automate across different browsers, Playwright might be your go-to.
Here's a real-world example: Trivago, the hotel search giant, switched from Puppeteer to Playwright for their testing. The result? They cut test execution time by 30% and had fewer flaky tests. That's a big win for their CI/CD pipeline.
But don't count Puppeteer out just yet. It's been around longer (since 2017) and has a bigger community. If you're working on Chrome-specific tasks, Puppeteer might still be your best bet.
Let's break it down:
Feature | Playwright | Puppeteer |
---|---|---|
Browser Support | Chrome, Firefox, WebKit | Mainly Chrome/Chromium |
Language Support | JavaScript, TypeScript, Python, Java, C# | JavaScript, TypeScript |
GitHub Stars (Jan 2024) | 58k | 85.7k |
Best For | Cross-browser stuff, complex scenarios | Chrome-focused projects, simpler tasks |
So, which one's better? It all comes down to what you need. Multi-browser testing? Playwright might be your jam. Chrome-specific automation? Puppeteer's got your back.